useStyles
This hook is used to apply shared effects/styles to elements. It is used internally by other components.
Anatomy
import { useStyles } from "@lualtek/react-components";
const MyComponent = (props) => {
const { vibrancy } = useStyles();
return (
<div {...vibrancy.attributes}>
Apply default vibrancy style and add elevation shadow
</div>
);
};
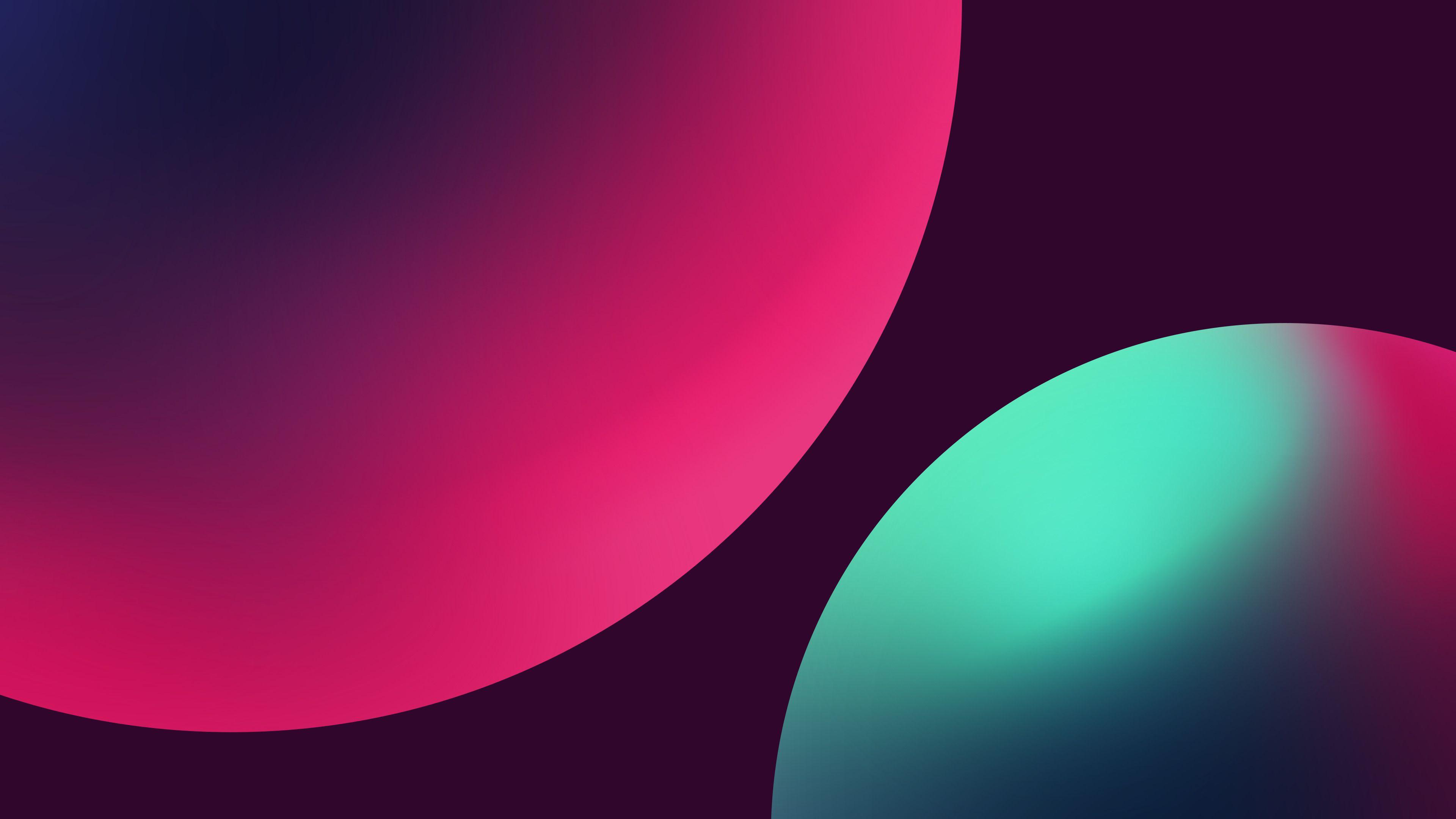
Apply default vibrancy style
Advanced usage
import { useStyles } from "@lualtek/react-components";
const MyComponent = (props) => {
/**
* You can pass an object to override the default values
*/
const { vibrancy, elevation } = useStyles({
elevation: {
resting: 2,
onHover: 4,
},
vibrancy: {
color: "soft",
saturation: "high",
},
});
return (
<div
style={{ ...elevation.style }}
{...elevation.attributes}
{...vibrancy.attributes}
>
Apply default vibrancy style and add elevation shadow
</div>
);
};
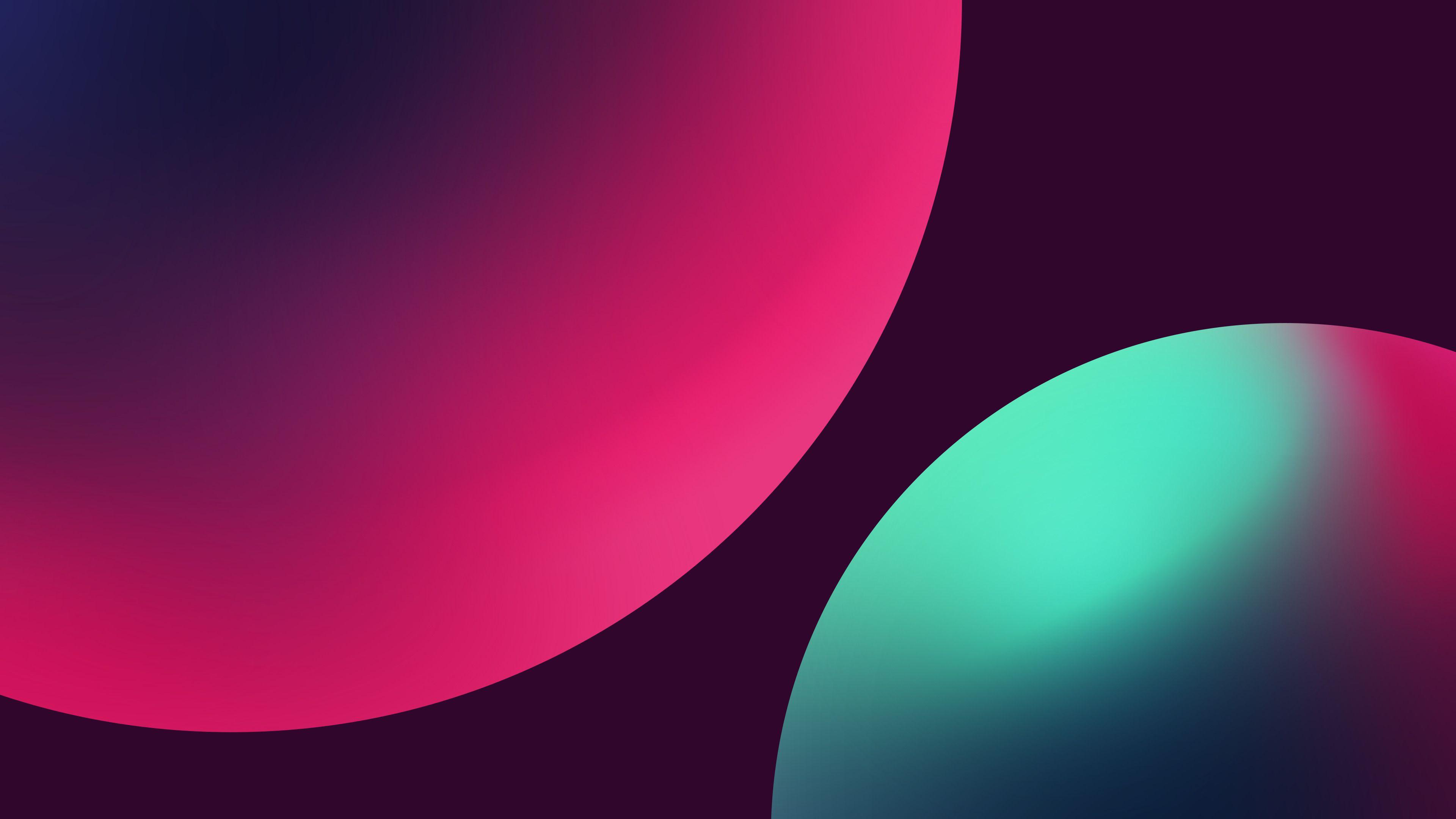
Apply vibrancy style and add elevation shadow
API Reference
⚠️
You should use this hook only when the Panel
component doesn't fit.
type UseStylesParams = {
vibrancy?: {
blur?: "soft" | "strong";
color?: "background" | "soft" | "mid" | "hard";
saturation?: "standard" | "high";
};
elevation?: {
resting?: 0 | 1 | 2 | 3 | 4;
onHover?: 0 | 1 | 2 | 3 | 4;
direction?: "right" | "left" | "top" | "bottom";
shadowColor?: string;
};
};